目錄
- 一.準(zhǔn)備數(shù)據(jù)
- 創(chuàng)建數(shù)據(jù)表
- 插入數(shù)據(jù)
- 二.SQL演練
- 1. SQL語句的強(qiáng)化
- 2. 創(chuàng)建 "商品分類"" 表
- 3. 同步表數(shù)據(jù)
- 4. 創(chuàng)建 "商品品牌表" 表
- 5. 同步數(shù)據(jù)
- 6. 修改表結(jié)構(gòu)
- 7. 外鍵
- 三.數(shù)據(jù)庫的設(shè)計(jì)
- 創(chuàng)建 "商品分類" 表(之前已經(jīng)創(chuàng)建,無需再次創(chuàng)建)
- 創(chuàng)建 "商品品牌" 表(之前已經(jīng)創(chuàng)建,無需再次創(chuàng)建)
- 創(chuàng)建 "商品" 表(之前已經(jīng)創(chuàng)建,無需再次創(chuàng)建)
- 創(chuàng)建 "顧客" 表
- 創(chuàng)建 "訂單" 表
- 創(chuàng)建 "訂單詳情" 表
- 說明
- 四.Python 中操作 MySQL 步驟
- 引入模塊
- Connection 對象
- Cursor對象
- 五.增刪改查
- 查詢一行數(shù)據(jù)
- 查詢多行數(shù)據(jù)
- 六.參數(shù)化
一.準(zhǔn)備數(shù)據(jù)
創(chuàng)建數(shù)據(jù)表
-- 創(chuàng)建 "京東" 數(shù)據(jù)庫
create database jing_dong charset=utf8;
-- 使用 "京東" 數(shù)據(jù)庫
use jing_dong;
-- 創(chuàng)建一個(gè)商品goods數(shù)據(jù)表
create table goods(
id int unsigned primary key auto_increment not null,
name varchar(150) not null,
cate_name varchar(40) not null,
brand_name varchar(40) not null,
price decimal(10,3) not null default 0,
is_show bit not null default 1,
is_saleoff bit not null default 0
);
插入數(shù)據(jù)
-- 向goods表中插入數(shù)據(jù)
insert into goods values(0,'r510vc 15.6英寸筆記本','筆記本','華碩','3399',default,default);
insert into goods values(0,'y400n 14.0英寸筆記本電腦','筆記本','聯(lián)想','4999',default,default);
insert into goods values(0,'g150th 15.6英寸游戲本','游戲本','雷神','8499',default,default);
insert into goods values(0,'x550cc 15.6英寸筆記本','筆記本','華碩','2799',default,default);
insert into goods values(0,'x240 超極本','超級本','聯(lián)想','4880',default,default);
insert into goods values(0,'u330p 13.3英寸超極本','超級本','聯(lián)想','4299',default,default);
insert into goods values(0,'svp13226scb 觸控超極本','超級本','索尼','7999',default,default);
insert into goods values(0,'ipad mini 7.9英寸平板電腦','平板電腦','蘋果','1998',default,default);
insert into goods values(0,'ipad air 9.7英寸平板電腦','平板電腦','蘋果','3388',default,default);
insert into goods values(0,'ipad mini 配備 retina 顯示屏','平板電腦','蘋果','2788',default,default);
insert into goods values(0,'ideacentre c340 20英寸一體電腦 ','臺式機(jī)','聯(lián)想','3499',default,default);
insert into goods values(0,'vostro 3800-r1206 臺式電腦','臺式機(jī)','戴爾','2899',default,default);
insert into goods values(0,'imac me086ch/a 21.5英寸一體電腦','臺式機(jī)','蘋果','9188',default,default);
insert into goods values(0,'at7-7414lp 臺式電腦 linux )','臺式機(jī)','宏碁','3699',default,default);
insert into goods values(0,'z220sff f4f06pa工作站','服務(wù)器/工作站','惠普','4288',default,default);
insert into goods values(0,'poweredge ii服務(wù)器','服務(wù)器/工作站','戴爾','5388',default,default);
insert into goods values(0,'mac pro專業(yè)級臺式電腦','服務(wù)器/工作站','蘋果','28888',default,default);
insert into goods values(0,'hmz-t3w 頭戴顯示設(shè)備','筆記本配件','索尼','6999',default,default);
insert into goods values(0,'商務(wù)雙肩背包','筆記本配件','索尼','99',default,default);
insert into goods values(0,'x3250 m4機(jī)架式服務(wù)器','服務(wù)器/工作站','ibm','6888',default,default);
insert into goods values(0,'商務(wù)雙肩背包','筆記本配件','索尼','99',default,default);
二.SQL演練
1. SQL語句的強(qiáng)化
查詢類型cate_name為 '超極本' 的商品名稱、價(jià)格
select name,price from goods where cate_name = '超級本';
顯示商品的種類
select cate_name from goods group by cate_name;
求所有電腦產(chǎn)品的平均價(jià)格,并且保留兩位小數(shù)
select round(avg(price),2) as avg_price from goods;
顯示每種商品的平均價(jià)格
select cate_name,avg(price) from goods group by cate_name;
查詢每種類型的商品中 最貴、最便宜、平均價(jià)、數(shù)量
select cate_name,max(price),min(price),avg(price),count(*) from goods group by cate_name;
查詢所有價(jià)格大于平均價(jià)格的商品,并且按價(jià)格降序排序
select id,name,price from goods
where price > (select round(avg(price),2) as avg_price from goods)
order by price desc;
查詢每種類型中最貴的電腦信息
select * from goods
inner join
(
select
cate_name,
max(price) as max_price,
min(price) as min_price,
avg(price) as avg_price,
count(*) from goods group by cate_name
) as goods_new_info
on goods.cate_name=goods_new_info.cate_name and goods.price=goods_new_info.max_price;
2. 創(chuàng)建 "商品分類"" 表
-- 創(chuàng)建商品分類表
create table if not exists goods_cates(
id int unsigned primary key auto_increment,
name varchar(40) not null
);
查詢goods表中商品的種類
select cate_name from goods group by cate_name;
將分組結(jié)果寫入到goods_cates數(shù)據(jù)表
insert into goods_cates (name) select cate_name from goods group by cate_name;
3. 同步表數(shù)據(jù)
通過goods_cates數(shù)據(jù)表來更新goods表
update goods as g inner join goods_cates as c on g.cate_name=c.name set g.cate_name=c.id;
4. 創(chuàng)建 "商品品牌表" 表
通過create...select來創(chuàng)建數(shù)據(jù)表并且同時(shí)寫入記錄,一步到位
-- select brand_name from goods group by brand_name;
-- 在創(chuàng)建數(shù)據(jù)表的時(shí)候一起插入數(shù)據(jù)
-- 注意: 需要對brand_name 用as起別名,否則name字段就沒有值
create table goods_brands (
id int unsigned primary key auto_increment,
name varchar(40) not null) select brand_name as name from goods group by brand_name;
5. 同步數(shù)據(jù)
通過goods_brands數(shù)據(jù)表來更新goods數(shù)據(jù)表
update goods as g inner join goods_brands as b on g.brand_name=b.name set g.brand_name=b.id;
6. 修改表結(jié)構(gòu)
查看 goods 的數(shù)據(jù)表結(jié)構(gòu),會(huì)發(fā)現(xiàn) cate_name 和 brand_name對應(yīng)的類型為 varchar 但是存儲(chǔ)的都是數(shù)字
通過alter table語句修改表結(jié)構(gòu)
alter table goods
change cate_name cate_id int unsigned not null,
change brand_name brand_id int unsigned not null;
7. 外鍵
分別在 goods_cates 和 goods_brands表中插入記錄
insert into goods_cates(name) values ('路由器'),('交換機(jī)'),('網(wǎng)卡');
insert into goods_brands(name) values ('海爾'),('清華同方'),('神舟');
在 goods 數(shù)據(jù)表中寫入任意記錄
insert into goods (name,cate_id,brand_id,price)
values('LaserJet Pro P1606dn 黑白激光打印機(jī)', 12, 4,'1849');
查詢所有商品的詳細(xì)信息 (通過內(nèi)連接)
select g.id,g.name,c.name,b.name,g.price from goods as g
inner join goods_cates as c on g.cate_id=c.id
inner join goods_brands as b on g.brand_id=b.id;
查詢所有商品的詳細(xì)信息 (通過左連接)
select g.id,g.name,c.name,b.name,g.price from goods as g
left join goods_cates as c on g.cate_id=c.id
left join goods_brands as b on g.brand_id=b.id;
- 如何防止無效信息的插入,就是可以在插入前判斷類型或者品牌名稱是否存在呢? 可以使用之前講過的外鍵來解決
- 外鍵約束:對數(shù)據(jù)的有效性進(jìn)行驗(yàn)證
- 關(guān)鍵字: foreign key,只有 innodb數(shù)據(jù)庫引擎 支持外鍵約束
- 對于已經(jīng)存在的數(shù)據(jù)表 如何更新外鍵約束
-- 給brand_id 添加外鍵約束成功
alter table goods add foreign key (brand_id) references goods_brands(id);
-- 給cate_id 添加外鍵失敗
-- 會(huì)出現(xiàn)1452錯(cuò)誤
-- 錯(cuò)誤原因:已經(jīng)添加了一個(gè)不存在的cate_id值12,因此需要先刪除
alter table goods add foreign key (cate_id) references goods_cates(id);
- 如何在創(chuàng)建數(shù)據(jù)表的時(shí)候就設(shè)置外鍵約束呢?
- 注意: goods 中的 cate_id 的類型一定要和 goods_cates 表中的 id 類型一致
create table goods(
id int primary key auto_increment not null,
name varchar(40) default '',
price decimal(5,2),
cate_id int unsigned,
brand_id int unsigned,
is_show bit default 1,
is_saleoff bit default 0,
foreign key(cate_id) references goods_cates(id),
foreign key(brand_id) references goods_brands(id)
);
如何取消外鍵約束
-- 需要先獲取外鍵約束名稱,該名稱系統(tǒng)會(huì)自動(dòng)生成,可以通過查看表創(chuàng)建語句來獲取名稱
show create table goods;
-- 獲取名稱之后就可以根據(jù)名稱來刪除外鍵約束
alter table goods drop foreign key 外鍵名稱;
在實(shí)際開發(fā)中,很少會(huì)使用到外鍵約束,會(huì)極大的降低表更新的效率
三.數(shù)據(jù)庫的設(shè)計(jì)
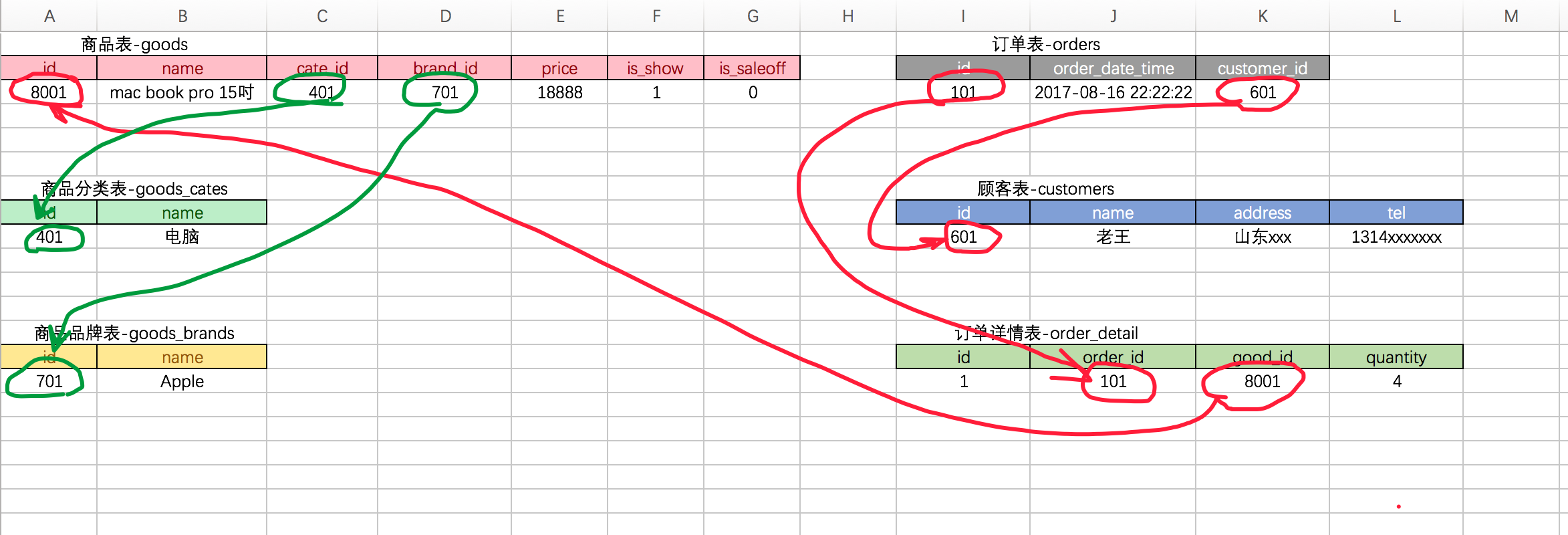
創(chuàng)建 "商品分類" 表(之前已經(jīng)創(chuàng)建,無需再次創(chuàng)建)
create table goods_cates(
id int unsigned primary key auto_increment not null,
name varchar(40) not null
);
創(chuàng)建 "商品品牌" 表(之前已經(jīng)創(chuàng)建,無需再次創(chuàng)建)
create table goods_brands (
id int unsigned primary key auto_increment not null,
name varchar(40) not null
);
創(chuàng)建 "商品" 表(之前已經(jīng)創(chuàng)建,無需再次創(chuàng)建)
create table goods(
id int unsigned primary key auto_increment not null,
name varchar(40) default '',
price decimal(5,2),
cate_id int unsigned,
brand_id int unsigned,
is_show bit default 1,
is_saleoff bit default 0,
foreign key(cate_id) references goods_cates(id),
foreign key(brand_id) references goods_brands(id)
);
創(chuàng)建 "顧客" 表
create table customer(
id int unsigned auto_increment primary key not null,
name varchar(30) not null,
addr varchar(100),
tel varchar(11) not null
);
創(chuàng)建 "訂單" 表
create table orders(
id int unsigned auto_increment primary key not null,
order_date_time datetime not null,
customer_id int unsigned,
foreign key(customer_id) references customer(id)
);
創(chuàng)建 "訂單詳情" 表
create table order_detail(
id int unsigned auto_increment primary key not null,
order_id int unsigned not null,
goods_id int unsigned not null,
quantity tinyint unsigned not null,
foreign key(order_id) references orders(id),
foreign key(goods_id) references goods(id)
);
說明
- 以上創(chuàng)建表的順序是有要求的,即如果goods表中的外鍵約束用的是goods_cates或者是goods_brands,那么就應(yīng)該先創(chuàng)建這2個(gè)表,否則創(chuàng)建goods會(huì)失敗
- 創(chuàng)建外鍵時(shí),一定要注意類型要相同,否則失敗
四.Python 中操作 MySQL 步驟
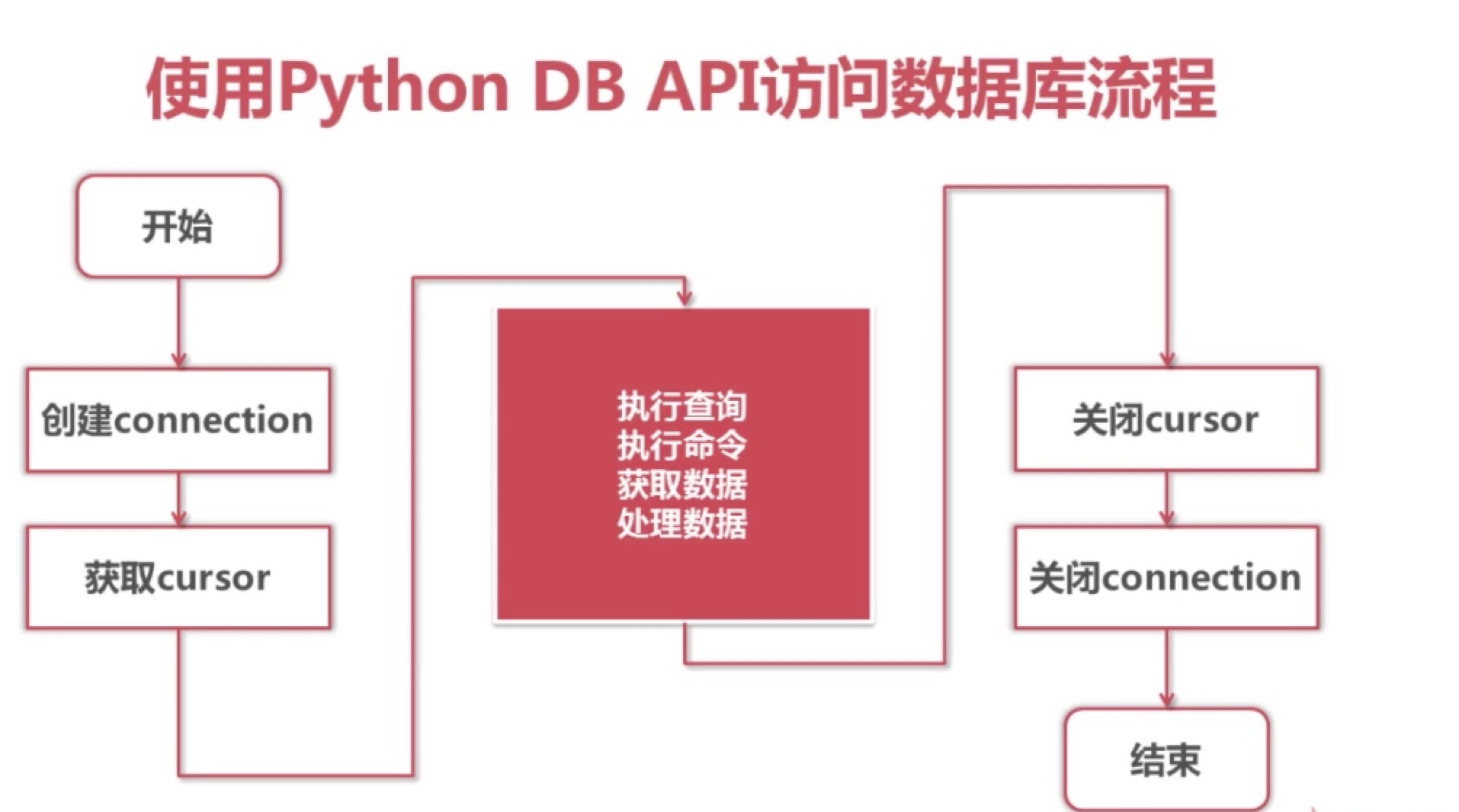
引入模塊
在py文件中引入pymysql模塊
Connection 對象
- 用于建立與數(shù)據(jù)庫的連接
- 創(chuàng)建對象:調(diào)用connect()方法
- 參數(shù)host:連接的mysql主機(jī),如果本機(jī)是'localhost'
- 參數(shù)port:連接的mysql主機(jī)的端口,默認(rèn)是3306
- 參數(shù)database:數(shù)據(jù)庫的名稱
- 參數(shù)user:連接的用戶名
- 參數(shù)password:連接的密碼
- 參數(shù)charset:通信采用的編碼方式,推薦使用utf8
對象的方法
- close()關(guān)閉連接
- commit()提交
- cursor()返回Cursor對象,用于執(zhí)行sql語句并獲得結(jié)果
Cursor對象
- 用于執(zhí)行sql語句,使用頻度最高的語句為select、insert、update、delete
- 獲取Cursor對象:調(diào)用Connection對象的cursor()方法
對象的方法
- close()關(guān)閉
- execute(operation [, parameters ])執(zhí)行語句,返回受影響的行數(shù),主要用于執(zhí)行insert、update、delete語句,也可以執(zhí)行create、alter、drop等語句
- fetchone()執(zhí)行查詢語句時(shí),獲取查詢結(jié)果集的第一個(gè)行數(shù)據(jù),返回一個(gè)元組
- fetchall()執(zhí)行查詢時(shí),獲取結(jié)果集的所有行,一行構(gòu)成一個(gè)元組,再將這些元組裝入一個(gè)元組返回
對象的屬性
- rowcount只讀屬性,表示最近一次execute()執(zhí)行后受影響的行數(shù)
- connection獲得當(dāng)前連接對象
五.增刪改查
from pymysql import *
def main():
# 創(chuàng)建Connection連接
conn = connect(host='localhost',port=3306,database='jing_dong',user='root',password='mysql',charset='utf8')
# 獲得Cursor對象
cs1 = conn.cursor()
# 執(zhí)行insert語句,并返回受影響的行數(shù):添加一條數(shù)據(jù)
# 增加
count = cs1.execute('insert into goods_cates(name) values("硬盤")')
#打印受影響的行數(shù)
print(count)
count = cs1.execute('insert into goods_cates(name) values("光盤")')
print(count)
# # 更新
# count = cs1.execute('update goods_cates set name="機(jī)械硬盤" where name="硬盤"')
# # 刪除
# count = cs1.execute('delete from goods_cates where id=6')
# 提交之前的操作,如果之前已經(jīng)之執(zhí)行過多次的execute,那么就都進(jìn)行提交
conn.commit()
# 關(guān)閉Cursor對象
cs1.close()
# 關(guān)閉Connection對象
conn.close()
if __name__ == '__main__':
main()
查詢一行數(shù)據(jù)
from pymysql import *
def main():
# 創(chuàng)建Connection連接
conn = connect(host='localhost',port=3306,user='root',password='mysql',database='jing_dong',charset='utf8')
# 獲得Cursor對象
cs1 = conn.cursor()
# 執(zhí)行select語句,并返回受影響的行數(shù):查詢一條數(shù)據(jù)
count = cs1.execute('select id,name from goods where id>=4')
# 打印受影響的行數(shù)
print("查詢到%d條數(shù)據(jù):" % count)
for i in range(count):
# 獲取查詢的結(jié)果
result = cs1.fetchone()
# 打印查詢的結(jié)果
print(result)
# 獲取查詢的結(jié)果
# 關(guān)閉Cursor對象
cs1.close()
conn.close()
if __name__ == '__main__':
main()
查詢多行數(shù)據(jù)
from pymysql import *
def main():
# 創(chuàng)建Connection連接
conn = connect(host='localhost',port=3306,user='root',password='mysql',database='jing_dong',charset='utf8')
# 獲得Cursor對象
cs1 = conn.cursor()
# 執(zhí)行select語句,并返回受影響的行數(shù):查詢一條數(shù)據(jù)
count = cs1.execute('select id,name from goods where id>=4')
# 打印受影響的行數(shù)
print("查詢到%d條數(shù)據(jù):" % count)
# for i in range(count):
# # 獲取查詢的結(jié)果
# result = cs1.fetchone()
# # 打印查詢的結(jié)果
# print(result)
# # 獲取查詢的結(jié)果
result = cs1.fetchall()
print(result)
# 關(guān)閉Cursor對象
cs1.close()
conn.close()
if __name__ == '__main__':
main()
六.參數(shù)化
- sql語句的參數(shù)化,可以有效防止sql注入
- 注意:此處不同于python的字符串格式化,全部使用%s占位
from pymysql import *
def main():
find_name = input("請輸入物品名稱:")
# 創(chuàng)建Connection連接
conn = connect(host='localhost',port=3306,user='root',password='mysql',database='jing_dong',charset='utf8')
# 獲得Cursor對象
cs1 = conn.cursor()
# # 非安全的方式
# # 輸入 " or 1=1 or " (雙引號也要輸入)
# sql = 'select * from goods where name="%s"' % find_name
# print("""sql===>%s====""" % sql)
# # 執(zhí)行select語句,并返回受影響的行數(shù):查詢所有數(shù)據(jù)
# count = cs1.execute(sql)
# 安全的方式
# 構(gòu)造參數(shù)列表
params = [find_name]
# 執(zhí)行select語句,并返回受影響的行數(shù):查詢所有數(shù)據(jù)
count = cs1.execute('select * from goods where name=%s', params)
# 注意:
# 如果要是有多個(gè)參數(shù),需要進(jìn)行參數(shù)化
# 那么params = [數(shù)值1, 數(shù)值2....],此時(shí)sql語句中有多個(gè)%s即可
# 打印受影響的行數(shù)
print(count)
# 獲取查詢的結(jié)果
# result = cs1.fetchone()
result = cs1.fetchall()
# 打印查詢的結(jié)果
print(result)
# 關(guān)閉Cursor對象
cs1.close()
# 關(guān)閉Connection對象
conn.close()
if __name__ == '__main__':
main()
以上就是MySQL和Python交互的示例的詳細(xì)內(nèi)容,更多關(guān)于MySQL和python交互的資料請關(guān)注腳本之家其它相關(guān)文章!
您可能感興趣的文章:- Python爬蟲爬取全球疫情數(shù)據(jù)并存儲(chǔ)到mysql數(shù)據(jù)庫的步驟
- Python爬取騰訊疫情實(shí)時(shí)數(shù)據(jù)并存儲(chǔ)到mysql數(shù)據(jù)庫的示例代碼
- 配置python連接oracle讀取excel數(shù)據(jù)寫入數(shù)據(jù)庫的操作流程
- Python 對Excel求和、合并居中的操作
- 如何用python合并多個(gè)excel文件
- python基于pyppeteer制作PDF文件
- python 實(shí)現(xiàn)存儲(chǔ)數(shù)據(jù)到txt和pdf文檔及亂碼問題的解決
- python操作mysql、excel、pdf的示例