目錄
- 1.準備工作
- 2. 開始
- 2.1 生成控件
- 2.2 定義輸入和計算函數(shù)
- 2.3 綁定鍵盤事件
- 2.4 循環(huán)
- 3.全部代碼
- 4. 結(jié)束語
做一個計算器,這是我想要達成的效果:
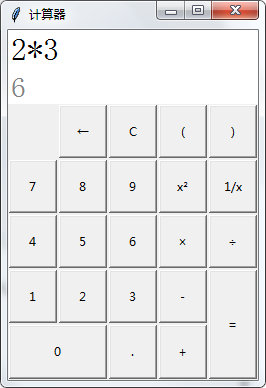
在按下按鈕或者按下鍵盤的時候,第一行輸入框會顯示輸入的內(nèi)容,第二行顯示框則會預覽運算結(jié)果,如果發(fā)生異常,輸入內(nèi)容格式錯誤,無法計算,則顯示框顯示“錯誤”。
按“=”按鈕或按鍵回車計算結(jié)果,結(jié)果顯示在第一行。
1.準備工作
導入庫 tkinter
2. 開始
定義兩個變量:
equal_is=False #定義一些變量
textchange=''
equal_is 用于判斷是否已經(jīng)計算出結(jié)果,textchange是用于設(shè)置輸入框的內(nèi)容。
2.1 生成控件
首先生成窗體:
root=tk.Tk() #創(chuàng)建窗體
root.geometry('250x350')
root.title('計算器')
為了方便,在這里定義一個函數(shù) create_btn
def create_btn(text,col,row,cs,rs,pri='',px=(1,1),py=(1,1)): #函數(shù):生成按鈕
if pri=='':
t=text
else:
t=pri
a=tk.Button(root,text=text,width=4,command=lambda:(text_print(t))) #輸入內(nèi)容
a.grid(column=col,row=row,columnspan=cs,rowspan=rs,padx=px,pady=py,sticky='nswe')
return(a)
因為有些按鈕的輸入內(nèi)容并不等于它的文本內(nèi)容(比如按鈕“×”,輸入“*”),因此我們設(shè)置一個空的參數(shù) pri ,在 pri 沒有被賦值的時候,輸入內(nèi)容則為 text 。
text_print 是輸入內(nèi)容的函數(shù),這個在后面會定義。
btn={} #生成按鈕
btn['1']=create_btn('1',0,5,1,1)
btn['2']=create_btn('2',1,5,1,1)
btn['3']=create_btn('3',2,5,1,1)
btn['4']=create_btn('4',0,4,1,1)
btn['5']=create_btn('5',1,4,1,1)
btn['6']=create_btn('6',2,4,1,1)
btn['7']=create_btn('7',0,3,1,1)
btn['8']=create_btn('8',1,3,1,1)
btn['9']=create_btn('9',2,3,1,1)
btn['0']=create_btn('0',0,6,2,1)
btn['.']=create_btn('.',2,6,1,1)
btn['=']=create_btn('=',4,5,1,2)
btn['+']=create_btn('+',3,6,1,1)
btn['-']=create_btn('-',3,5,1,1)
btn['*']=create_btn('×',3,4,1,1,pri='*')
btn['/']=create_btn('÷',4,4,1,1,pri='/')
btn['←']=create_btn('←',1,2,1,1)
btn['C']=create_btn('C',2,2,1,1)
btn['(']=create_btn('(',3,2,1,1)
btn[')']=create_btn(')',4,2,1,1)
btn['**2']=create_btn('x²',3,3,1,1,pri='**2')
btn['**(-1)']=create_btn('1/x',4,3,1,1,pri='**(-1)')
上面是用 create_btn 函數(shù)生成每一個按鈕。
la=tk.Label(root,text='',bg='white',fg='black',font=('宋體',24),anchor='w',relief='flat') #生成輸入框
la.grid(column=0,row=0,columnspan=5,rowspan=1,sticky='we')
lab=tk.Label(root,bg='white',fg='grey',height=1,font=('宋體',22),anchor='w',relief='flat') #生成顯示框
lab.grid(column=0,row=1,columnspan=5,rowspan=1,sticky='we')
上面創(chuàng)建兩個標簽,作為輸入框和顯示框。
la 是輸入框,lab是顯示框。
然后定義函數(shù) grid_rowconfigure 和 grid_columnconfigure,用于自動填充行和列:
def grid_rowconfigure(*rows): #函數(shù)填充行。*rows:允許接收多個參數(shù)
for i in rows:
root.grid_rowconfigure(i,weight=1)
def grid_columnconfigure(*cols): #函數(shù)填充列。*cols:允許接收多個參數(shù)
for i in cols:
root.grid_columnconfigure(i,weight=1)
在窗體被改變大小時,按鈕會自動填充四周,而輸入、顯示框只填充左右兩邊(第2,3,4,5,6行會向豎直方向填充,每一列都會向水平方向填充)。
grid_rowconfigure(2,3,4,5,6)
grid_columnconfigure(0,1,2,3,4)
2.2 定義輸入和計算函數(shù)
定義 text_print 函數(shù),當按鈕被點擊時輸入內(nèi)容,當按鈕“=”被點擊的時候計算結(jié)果:
def text_print(x): #函數(shù)按鈕輸入算式
global textchange,equal_is #聲明全局變量
if x!='=':
if x=='←':
a=str(textchange)[0:-1]
textchange=a #退格
elif x=='C':
textchange='' #清空
else:
textchange=str(textchange)+str(x) #輸入
la.configure(text=textchange)
show_is()
equal_is=False #判斷格式有無錯誤
if x=='=':
text_equal() #計算結(jié)果
show_is 用于判斷格式有無錯誤:
def show_is(): #顯示框內(nèi)容
global textchange #聲明全局變量
if textchange!='':
try:
textshow=eval(textchange)
except (SyntaxError,TypeError,NameError):
lab.configure(text='錯誤') #如果出錯了,則顯示“錯誤”
else:
lab.configure(text=textshow) #如果沒有出錯,則顯示結(jié)果
else:
lab.configure(text='') #如果輸入框為空,那么清空顯示框
text_equal 是計算結(jié)果的函數(shù):
def text_equal(event=None): #函數(shù)計算結(jié)果并上到輸入框
global textchange,equal_is #聲明全局變量
if lab['text']!='錯誤' and equal_is==False:
textchange=lab['text'] #無格式錯誤時,計算結(jié)果
la.configure(text=textchange) #輸入框顯示結(jié)果
lab.configure(text='') #清空顯示框
equal_is=True
這里詳細說明一下 equal_is 的作用:
因為計算了結(jié)果后顯示框會清空,為了防止二次計算導致輸入框也跟著清空,我們需要判斷是否已經(jīng)計算過結(jié)果,這時就用到變量 equal_is 。
當輸入內(nèi)容時,equal_is 變?yōu)?True,計算結(jié)果后,equal_is 變?yōu)?False,如果 equal_is == True,則不計算結(jié)果。
2.3 綁定鍵盤事件
我設(shè)置的鍵盤按鍵及其對應輸入內(nèi)容:
輸入內(nèi)容 |
對應按鍵 |
0~9 |
0~9 |
+ |
+ |
- |
- |
* |
* |
/ |
/ |
退格 |
BackSpace |
清空 |
Delete |
計算結(jié)果 |
Return(Enter鍵) |
定義一個函數(shù) bind_print,跟 text_print 有點相似,但有些不一樣(原諒我技術(shù)差,不知道別的方法,只能重新定義一個函數(shù)):
def bind_print(event): #函數(shù)鍵盤事件輸入算式
global textchange,equal_is
if event.keysym!='Return':
if event.keysym=='BackSpace': #如果按鍵名等于“BackSpace”(退格鍵),那么就退格
a=str(textchange)[0:-1]
textchange=a
elif event.keysym=='Delete': #清空
textchange=''
else:
textchange=str(textchange)+str(event.char) #輸入按鍵內(nèi)容,char不會獲得Ctrl,Shift等特殊按鍵的文本
la.configure(text=textchange) #顯示內(nèi)容
show_is() #判斷是否錯誤
equal_is=False
else:
text_equal()
如果按下的是特殊按鍵,除非是退格和回車,否則都不會有反應,
按下字母、數(shù)字、符號鍵的時候,輸入按鍵內(nèi)容。
接下來就是綁定鍵盤事件了:
root.bind('Key>',bind_print) #當鍵盤按下任意鍵,執(zhí)行bind_print
這樣,界面布置和功能就完成了‘
2.4 循環(huán)
將主窗體root放入主循環(huán)中:
3.全部代碼
import tkinter as tk
def create_btn(text,col,row,cs,rs,pri='',px=(1,1),py=(1,1)): #函數(shù)生成按鈕
if pri=='':
t=text
else:
t=pri
a=tk.Button(root,text=text,width=4,command=lambda:(text_print(t)))
a.grid(column=col,row=row,columnspan=cs,rowspan=rs,padx=px,pady=py,sticky='nswe')
return(a)
def grid_rowconfigure(*rows): #函數(shù)填充行
for i in rows:
root.grid_rowconfigure(i,weight=1)
def grid_columnconfigure(*cols): #函數(shù)填充列
for i in cols:
root.grid_columnconfigure(i,weight=1)
def bind_print(event): #函數(shù)鍵盤事件輸入算式
global textchange,equal_is
if event.keysym!='Return':
if event.keysym=='BackSpace':
a=str(textchange)[0:-1]
textchange=a
elif event.keysym=='Delete':
textchange=''
else:
textchange=str(textchange)+str(event.char)
la.configure(text=textchange)
show_is()
equal_is=False
else:
text_equal()
def text_print(x): #函數(shù)按鈕輸入算式
global textchange,equal_is
if x!='=':
if x=='←':
a=str(textchange)[0:-1]
textchange=a
elif x=='C':
textchange=''
else:
textchange=str(textchange)+str(x)
la.configure(text=textchange)
show_is()
equal_is=False
if x=='=':
text_equal()
def text_equal(event=None): #函數(shù)計算結(jié)果并上到輸入框
global textchange,equal_is
if lab['text']!='錯誤' and equal_is==False:
textchange=lab['text']
la.configure(text=textchange)
lab.configure(text='')
equal_is=True
def show_is(): #顯示框內(nèi)容
global textchange
if textchange!='':
try:
textshow=eval(textchange)
except (SyntaxError,TypeError,NameError):
lab.configure(text='錯誤')
else:
lab.configure(text=textshow)
else:
lab.configure(text='')
root=tk.Tk() #創(chuàng)建窗體
root.geometry('250x350')
root.title('計算器')
root.bind('Key>',bind_print)
equal_is=False #定義一些函數(shù)
textchange=''
la=tk.Label(root,text='',bg='white',fg='black',font=('宋體',24),anchor='w',relief='flat') #生成輸入框
la.grid(column=0,row=0,columnspan=5,rowspan=1,sticky='we')
lab=tk.Label(root,bg='white',fg='grey',height=1,font=('宋體',22),anchor='w',relief='flat') #生成顯示框
lab.grid(column=0,row=1,columnspan=5,rowspan=1,sticky='we')
btn={} #生成按鈕
btn['1']=create_btn('1',0,5,1,1)
btn['2']=create_btn('2',1,5,1,1)
btn['3']=create_btn('3',2,5,1,1)
btn['4']=create_btn('4',0,4,1,1)
btn['5']=create_btn('5',1,4,1,1)
btn['6']=create_btn('6',2,4,1,1)
btn['7']=create_btn('7',0,3,1,1)
btn['8']=create_btn('8',1,3,1,1)
btn['9']=create_btn('9',2,3,1,1)
btn['0']=create_btn('0',0,6,2,1)
btn['.']=create_btn('.',2,6,1,1)
btn['=']=create_btn('=',4,5,1,2)
btn['+']=create_btn('+',3,6,1,1)
btn['-']=create_btn('-',3,5,1,1)
btn['*']=create_btn('×',3,4,1,1,pri='*')
btn['/']=create_btn('÷',4,4,1,1,pri='/')
btn['←']=create_btn('←',1,2,1,1)
btn['C']=create_btn('C',2,2,1,1)
btn['(']=create_btn('(',3,2,1,1)
btn[')']=create_btn(')',4,2,1,1)
btn['**2']=create_btn('x²',3,3,1,1,pri='**2')
btn['**(-1)']=create_btn('1/x',4,3,1,1,pri='**(-1)')
grid_rowconfigure(2,3,4,5,6)
grid_columnconfigure(0,1,2,3,4)
root.mainloop()
4. 結(jié)束語
以上就是做一個簡單計算器的過程,效果如開頭所示。
本人技術(shù)還較差,歡迎向我提出任何的意見。
到此這篇關(guān)于如何利用python的tkinter實現(xiàn)一個簡單的計算器的文章就介紹到這了,更多相關(guān)python tkinter簡單計算器內(nèi)容請搜索腳本之家以前的文章或繼續(xù)瀏覽下面的相關(guān)文章希望大家以后多多支持腳本之家!
您可能感興趣的文章:- python基于tkinter制作下班倒計時工具
- Python實戰(zhàn)之用tkinter庫做一個鼠標模擬點擊器
- Python聊天室?guī)Ы缑鎸崿F(xiàn)的示例代碼(tkinter,Mysql,Treading,socket)
- python基于tkinter制作m3u8視頻下載工具
- Python中tkinter的用戶登錄管理的實現(xiàn)
- python tkinter實現(xiàn)定時關(guān)機
- python中Tkinter 窗口之輸入框和文本框的實現(xiàn)
- python Tkinter的簡單入門教程
- python tkinter 獲得按鈕的文本值
- Python基礎(chǔ)之tkinter圖形化界面學習