本文實例為大家分享了Jsp+Servlet實現(xiàn)簡單登錄注冊查詢的具體代碼,供大家參考,具體內(nèi)容如下
1、注冊功能:
制作一個注冊頁面
用戶輸入:
用戶名
密碼
年齡
注冊成功:——>跳轉(zhuǎn)至登錄頁面進(jìn)行登錄
注冊失敗:——>文字或其他形式的提示皆可
2、簡易查詢:
制作一個查詢頁面
輸入用戶名
顯示該用戶的用戶名、密碼、年齡
演示
1.啟動進(jìn)入登陸頁面
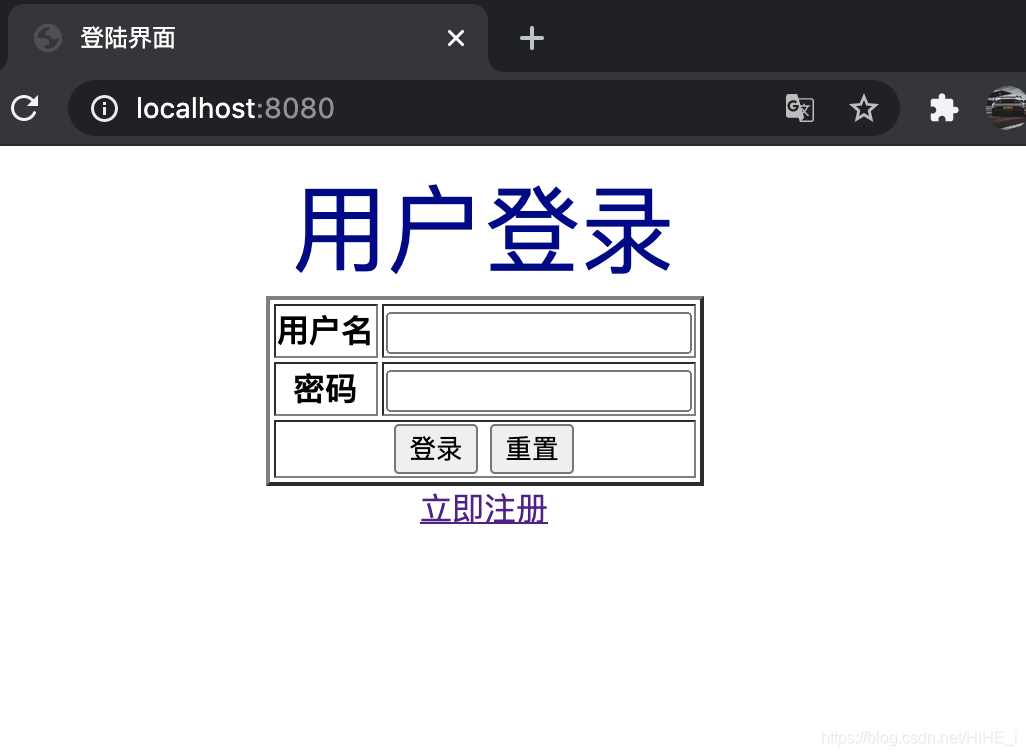
2.點擊注冊,進(jìn)入注冊頁面,成功跳轉(zhuǎn)到登錄頁面
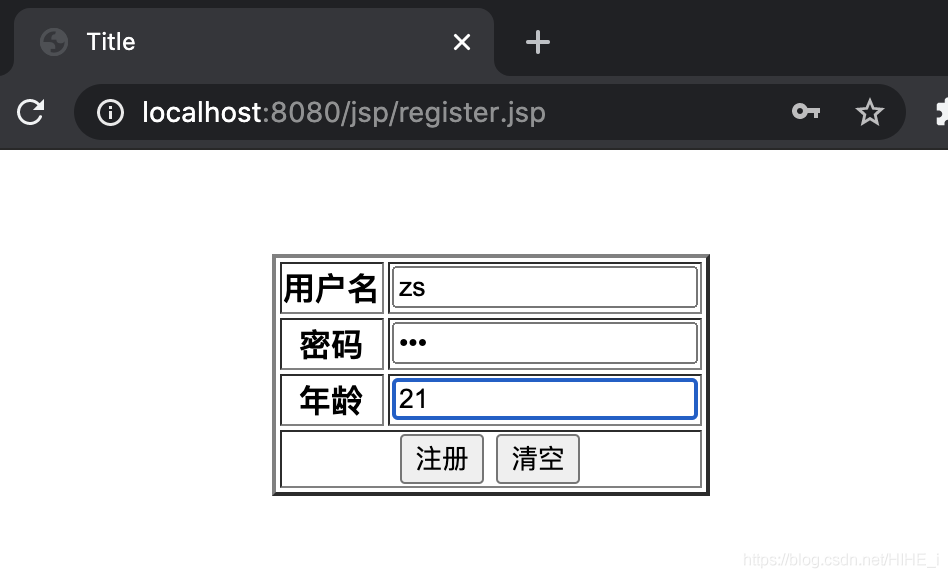
失敗則提示

回到登錄頁面,登錄成功進(jìn)入查詢頁面
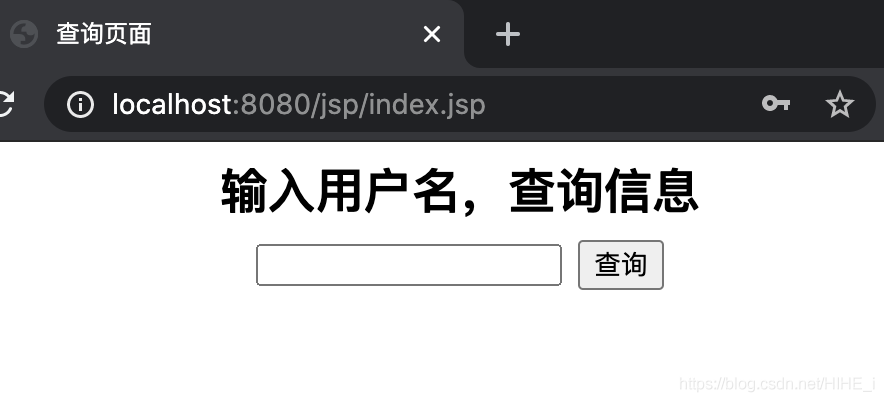
登錄失敗顯示提示信息

輸入用戶名->顯示該用戶的用戶名、密碼、年齡
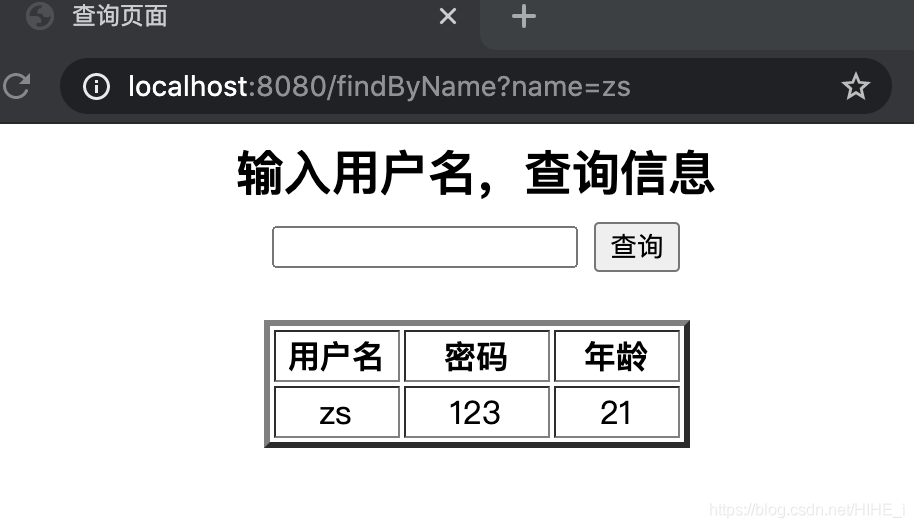
代碼
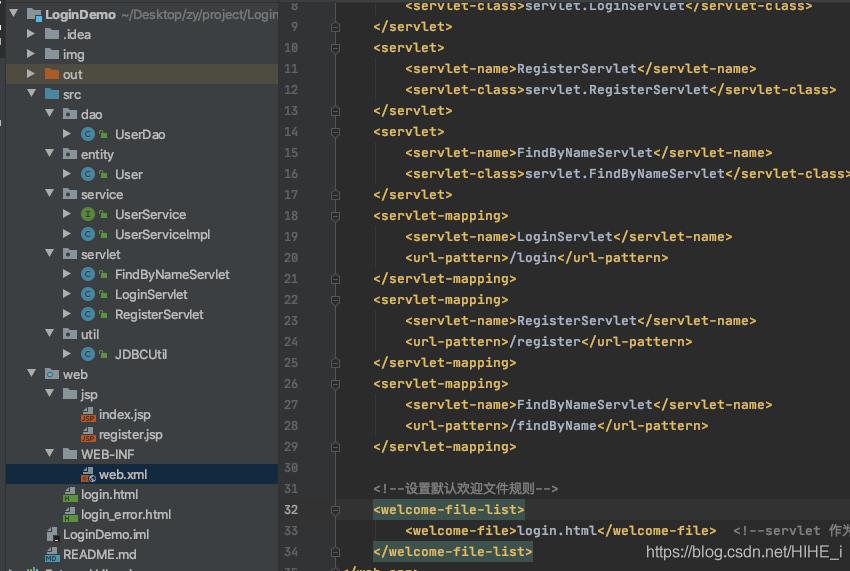
dao
public class UserDao {
private Connection conn = null;
private PreparedStatement ps=null;
private int result=0;
private ResultSet rs=null;
//用戶注冊
public int register(User user){
String sql="insert into users(name,password,age) value (?,?,?)";
try {
//獲取數(shù)據(jù)庫連接對象
conn= JDBCUtil.getConnection();
//獲取數(shù)據(jù)庫操作對象
ps=conn.prepareStatement(sql);
ps.setString(1,user.getName());
ps.setString(2,user.getPassword());
ps.setInt(3,user.getAge());
//執(zhí)行sql
result=ps.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
}finally {
JDBCUtil.close(null,ps,conn);
}
return result;
}
//登錄驗證用戶信息
public int login(String userName,String password){
String sql ="select count(*) from users where name=? and password=?";
try {
conn=JDBCUtil.getConnection();
ps=conn.prepareStatement(sql);
ps.setString(1,userName);
ps.setString(2,password);
rs=ps.executeQuery();
while (rs.next()){
result=rs.getInt("count(*)");
}
} catch (Exception e) {
e.printStackTrace();
} finally {
JDBCUtil.close(rs,ps,conn);
}
return result;
}
//根據(jù)用戶名 顯示用戶名、密碼、年齡
public User findByName(String userName){
String sql="select name,password,age from users where name=?";
User user = null;
try {
conn=JDBCUtil.getConnection();
ps=conn.prepareStatement(sql);
ps.setString(1,userName);
rs=ps.executeQuery();
while (rs.next()){
String name = rs.getString("name");
String password = rs.getString("password");
int age = rs.getInt("age");
user = new User(name,password,age);
}
} catch (Exception e) {
e.printStackTrace();
}finally {
JDBCUtil.close(null,ps,conn);
}
return user;
}
}
entity 實體類
public class User {
private int id;
private String name;
private String password;
private int age;
//set...
//get...
//constructor...
}
service
public class UserServiceImpl implements UserService {
UserDao userDao = new UserDao();
// 注冊
@Override
public int register(User user) {
return userDao.register(user);
}
// 登陸
@Override
public int login(String userName, String password) {
return userDao.login(userName,password);
}
// 根據(jù)用戶名查找信息
@Override
public User findByName(String userName) {
return userDao.findByName(userName);
}
}
servlet
// FindByNameServlet
public class FindByNameServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String name = request.getParameter("name");
UserService userService = new UserServiceImpl();
User user = userService.findByName(name);
//將查詢結(jié)果放入request作用域
request.setAttribute("userInfo",user);
request.getRequestDispatcher("/jsp/index.jsp").forward(request,response);
}
}
// LoginServlet
public class LoginServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//1 獲取
String userName = request.getParameter("userName");
String password = request.getParameter("password");
//2 service調(diào)用dao對數(shù)據(jù)庫操作
UserService userService = new UserServiceImpl();
int result = userService.login(userName, password);
//3 成功跳轉(zhuǎn)到查詢頁面,失敗跳轉(zhuǎn)到失敗頁面
if (result>0){
response.sendRedirect("/jsp/index.jsp");
}else{
response.sendRedirect("/login_error.html");
}
}
}
// RegisterServlet
public class RegisterServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
UserService userService = new UserServiceImpl();
User user = null;
int result = 0;
//1【調(diào)用請求對象】讀取【請求頭】參數(shù)信息,得到用戶注冊信息
String userName, password, age;
userName = request.getParameter("userName");
password = request.getParameter("password");
age = request.getParameter("age");
user = new User(userName, password, Integer.valueOf(age));
//2 調(diào)用userService——>userDao
// 先查詢用戶是否存在
User byName = userService.findByName(userName);
if (byName!=null){
request.setAttribute("info","用戶已存在!");
request.getRequestDispatcher("/jsp/register.jsp").forward(request,response);
}
// 注冊
result = userService.register(user);
//3 設(shè)置編碼格式,防止亂碼
response.setContentType("text/html;charset=utf-8");
PrintWriter out = response.getWriter();
//注冊成功:——>跳轉(zhuǎn)至登錄頁面進(jìn)行登錄
//注冊失?。骸?gt;注冊頁面提示:注冊失敗
if (result == 1) {
response.sendRedirect("/login.html");
} else {
request.setAttribute("info","注冊失??!");
request.getRequestDispatcher("/jsp/register.jsp").forward(request,response);
}
}
}
JDBCUtil
public class JDBCUtil {
private JDBCUtil(){}
//靜態(tài)代碼塊在類加載時執(zhí)行,并且執(zhí)行一次。
static{
try {
Class.forName("com.mysql.cj.jdbc.Driver");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
//獲取數(shù)據(jù)庫連接對象
public static Connection getConnection() throws Exception{
String url="jdbc:mysql://127.0.0.1:3306/zy?useSSL=falseserverTimezone=UTCrewriteBatchedStatements=true";
String user="root";
String password="rootroot";
return DriverManager.getConnection(url,user,password);
}
/**
*關(guān)閉資源
* @param conn 連接對象
* @param ps 數(shù)據(jù)庫操作對象
* @param rs 結(jié)果集
*/
public static void close(ResultSet rs, Statement ps, Connection conn){
if (rs != null) {
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (ps != null) {
try {
ps.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (conn != null) {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
index.jsp
%@ page import="entity.User" %>
%@ page contentType="text/html;charset=UTF-8" language="java" %>
html>
head>
title>查詢頁面/title>
/head>
body>
div align="center">
h2/>輸入用戶名,查詢信息
form action="/findByName" method="get">
input type="text" name="name" id="name">
input type="submit" value="查詢">
/form>
%
User userInfo = (User) request.getAttribute("userInfo");
%>
%
if (userInfo != null) {
%>
table border="3">
tr>
th>用戶名/th>
th>密碼/th>
th>年齡/th>
/tr>
tr>
td> nbsp; nbsp; %=userInfo.getName()%> nbsp; nbsp;/td>
td> nbsp; nbsp; %=userInfo.getPassword()%> nbsp; nbsp;/td>
td> nbsp; nbsp; %=userInfo.getAge()%> nbsp; nbsp;/td>
/tr>
/table>
%
}
%>
/div>
/body>
/html>
register.jsp
%@ page import="com.mysql.cj.util.StringUtils" %>
%@ page contentType="text/html;charset=UTF-8" language="java" %>
html>
head>
title>Title/title>
/head>
body>
br>
br>
%
String info =(String) request.getAttribute("info");
%>
%
if (!StringUtils.isNullOrEmpty(info)){
%>
h1 style="color: red;text-align: center" >%=info%>/h1>
%
}
%>
div align="center">
form action="/register" method="post">
table border="2">
tr>
th>用戶名/th>
td>input type="text" name="userName"/>/td>
/tr>
tr>
th>密碼/th>
td>input type="password" name="password"/>/td>
/tr>
tr>
th>年齡/th>
td>input type="text" name="age"/>/td>
/tr>
tr>
td colspan="2" align="center">
input type="submit" value="注冊"/>
input type="reset" value="清空"/>
/td>
/tr>
/table>
/form>
/div>
/body>
/html>
web.xml
?xml version="1.0" encoding="UTF-8"?>
web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
servlet>
servlet-name>LoginServlet/servlet-name>
servlet-class>servlet.LoginServlet/servlet-class>
/servlet>
servlet>
servlet-name>RegisterServlet/servlet-name>
servlet-class>servlet.RegisterServlet/servlet-class>
/servlet>
servlet>
servlet-name>FindByNameServlet/servlet-name>
servlet-class>servlet.FindByNameServlet/servlet-class>
/servlet>
servlet-mapping>
servlet-name>LoginServlet/servlet-name>
url-pattern>/login/url-pattern>
/servlet-mapping>
servlet-mapping>
servlet-name>RegisterServlet/servlet-name>
url-pattern>/register/url-pattern>
/servlet-mapping>
servlet-mapping>
servlet-name>FindByNameServlet/servlet-name>
url-pattern>/findByName/url-pattern>
/servlet-mapping>
!--設(shè)置默認(rèn)歡迎文件規(guī)則-->
welcome-file-list>
welcome-file>login.html/welcome-file> !--servlet 作為默認(rèn)歡迎文件 ‘/'需要去掉-->
/welcome-file-list>
/web-app>
login.html
!DOCTYPE html>
html lang="en">
head>
meta charset="UTF-8">
title>登陸界面/title>
/head>
body>
div align="center">
font size="10px" color="#00008b">用戶登錄/font>
form action="/login" method="post">
table border="2">
tr>
th>用戶名/th>
td>input type="text" name="userName"/>/td>
/tr>
tr>
th>密碼/th>
td>input type="password" name="password"/>/td>
/tr>
tr>
td colspan="2" align="center">
input type="submit" value="登錄"/>
input type="reset" />
/td>
/tr>
/table>
/form>
a href="/jsp/register.jsp" style="text-align: left">立即注冊/a>
/div>
/body>
/html>
login_error.html
!DOCTYPE html>
html lang="en">
head>
meta charset="UTF-8">
title>登錄驗證/title>
/head>
body>
div align="center">
font size="10px" color="#00008b">用戶登錄/font>br>
font size="5px" color="red">登錄信息不存在,請重新登陸?。?!/font>
form action="/login" method="post">
table border="2">
tr>
th>用戶名/th>
td>input type="text" name="userName" />/td>
/tr>
tr>
th>密碼/th>
td>input type="password" name="password" />/td>
/tr>
tr>
td colspan="2" align="center">
input type="submit" value="登錄"/>
input type="reset">
/td>
/tr>
/table>
/form>
a href="/jsp/register.jsp" style="text-align: left">立即注冊/a>
/div>
/body>
/html>
以上就是本文的全部內(nèi)容,希望對大家的學(xué)習(xí)有所幫助,也希望大家多多支持腳本之家。
您可能感興趣的文章:- JS pushlet XMLAdapter適配器用法案例解析
- 如何將JSP/Servlet項目轉(zhuǎn)換為Spring Boot項目
- JavaScript中ES6規(guī)范中l(wèi)et和const的用法和區(qū)別
- jsp學(xué)習(xí)之scriptlet的使用方法詳解
- jsp+servlet簡單實現(xiàn)上傳文件功能(保存目錄改進(jìn))
- 基于leaflet.js實現(xiàn)修改地圖主題樣式的流程分析
- leaflet加載geojson疊加顯示功能代碼
- JavaScript中l(wèi)et避免閉包造成問題