多邊形選區(qū)概述
多邊形選區(qū)是一種常見的對象選擇方式,在一個(gè)子圖中,單擊鼠標(biāo)左鍵即構(gòu)建一個(gè)多邊形的端點(diǎn),最后一個(gè)端點(diǎn)與第一個(gè)端點(diǎn)重合即完成多邊形選區(qū),選區(qū)即為多個(gè)端點(diǎn)構(gòu)成的多邊形。在matplotlib中的多邊形選區(qū)屬于部件(widgets),matplotlib中的部件都是中性(neutral )的,即與具體后端實(shí)現(xiàn)無關(guān)。
多邊形選區(qū)具體實(shí)現(xiàn)定義為matplotlib.widgets.PolygonSelector類,繼承關(guān)系為:Widget->AxesWidget->_SelectorWidget->PolygonSelector。
PolygonSelector類的簽名為class matplotlib.widgets.PolygonSelector(ax, onselect, useblit=False, lineprops=None, markerprops=None, vertex_select_radius=15)
PolygonSelector類構(gòu)造函數(shù)的參數(shù)為:
- ax:多邊形選區(qū)生效的子圖,類型為matplotlib.axes.Axes的實(shí)例。
- onselect:多邊形選區(qū)完成后執(zhí)行的回調(diào)函數(shù),函數(shù)簽名為def onselect( vertices),vertices數(shù)據(jù)類型為列表,列表元素格式為(xdata,ydata)元組。
- drawtype:多邊形選區(qū)的外觀,取值范圍為{"box", "line", "none"},"box"為多邊形框,"line"為多邊形選區(qū)對角線,"none"無外觀,類型為字符串,默認(rèn)值為"box"。
- lineprops:多邊形選區(qū)線條的屬性,默認(rèn)值為dict(color='k', linestyle='-', linewidth=2, alpha=0.5)。
- markerprops:多邊形選區(qū)端點(diǎn)的屬性,默認(rèn)值為dict(marker='o', markersize=7, mec='k', mfc='k', alpha=0.5)。
- vertex_select_radius:多邊形端點(diǎn)的選擇半徑,浮點(diǎn)數(shù),默認(rèn)值為15,用于端點(diǎn)選擇或者多邊形閉合。
PolygonSelector類中的state_modifier_keys公有變量 state_modifier_keys定義了操作快捷鍵,類型為字典。
- “move_all”: 移動已存在的選區(qū),默認(rèn)為"shift"。
- “clear”:清除現(xiàn)有選區(qū),默認(rèn)為 "escape",即esc鍵。
- “move_vertex”:正方形選區(qū),默認(rèn)為"control"。
PolygonSelector類中的verts特性返回多邊形選區(qū)中的多有端點(diǎn),類型為列表,元素為(x,y)元組,即端點(diǎn)的坐標(biāo)元組。
案例
官方案例,https://matplotlib.org/gallery/widgets/polygon_selector_demo.html
案例說明
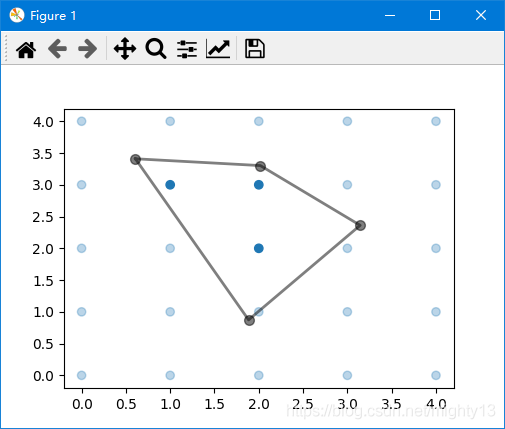
單擊鼠標(biāo)左鍵創(chuàng)建端點(diǎn),最終點(diǎn)擊初始端點(diǎn)閉合多邊形,形成多邊形選區(qū)。選區(qū)外的數(shù)據(jù)元素顏色變淡,選區(qū)內(nèi)數(shù)據(jù)顏色保持不變。
按esc鍵取消選區(qū)。按shift鍵鼠標(biāo)可以移動多邊形選區(qū)位置,按ctrl鍵鼠標(biāo)可以移動多邊形選區(qū)某個(gè)端點(diǎn)的位置。退出程序時(shí),控制臺輸出選區(qū)內(nèi)數(shù)據(jù)元素的坐標(biāo)。
控制臺輸出:
Selected points:
[[2.0 2.0]
[1.0 3.0]
[2.0 3.0]]
案例代碼
import numpy as np
from matplotlib.widgets import PolygonSelector
from matplotlib.path import Path
class SelectFromCollection:
"""
Select indices from a matplotlib collection using `PolygonSelector`.
Selected indices are saved in the `ind` attribute. This tool fades out the
points that are not part of the selection (i.e., reduces their alpha
values). If your collection has alpha 1, this tool will permanently
alter the alpha values.
Note that this tool selects collection objects based on their *origins*
(i.e., `offsets`).
Parameters
----------
ax : `~matplotlib.axes.Axes`
Axes to interact with.
collection : `matplotlib.collections.Collection` subclass
Collection you want to select from.
alpha_other : 0 = float = 1
To highlight a selection, this tool sets all selected points to an
alpha value of 1 and non-selected points to *alpha_other*.
"""
def __init__(self, ax, collection, alpha_other=0.3):
self.canvas = ax.figure.canvas
self.collection = collection
self.alpha_other = alpha_other
self.xys = collection.get_offsets()
self.Npts = len(self.xys)
# Ensure that we have separate colors for each object
self.fc = collection.get_facecolors()
if len(self.fc) == 0:
raise ValueError('Collection must have a facecolor')
elif len(self.fc) == 1:
self.fc = np.tile(self.fc, (self.Npts, 1))
self.poly = PolygonSelector(ax, self.onselect)
self.ind = []
def onselect(self, verts):
path = Path(verts)
self.ind = np.nonzero(path.contains_points(self.xys))[0]
self.fc[:, -1] = self.alpha_other
self.fc[self.ind, -1] = 1
self.collection.set_facecolors(self.fc)
self.canvas.draw_idle()
def disconnect(self):
self.poly.disconnect_events()
self.fc[:, -1] = 1
self.collection.set_facecolors(self.fc)
self.canvas.draw_idle()
if __name__ == '__main__':
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
grid_size = 5
grid_x = np.tile(np.arange(grid_size), grid_size)
grid_y = np.repeat(np.arange(grid_size), grid_size)
pts = ax.scatter(grid_x, grid_y)
selector = SelectFromCollection(ax, pts)
print("Select points in the figure by enclosing them within a polygon.")
print("Press the 'esc' key to start a new polygon.")
print("Try holding the 'shift' key to move all of the vertices.")
print("Try holding the 'ctrl' key to move a single vertex.")
plt.show()
selector.disconnect()
# After figure is closed print the coordinates of the selected points
print('\nSelected points:')
print(selector.xys[selector.ind])
到此這篇關(guān)于matplotlib之多邊形選區(qū)(PolygonSelector)的使用的文章就介紹到這了,更多相關(guān)matplotlib 多邊形選區(qū)內(nèi)容請搜索腳本之家以前的文章或繼續(xù)瀏覽下面的相關(guān)文章希望大家以后多多支持腳本之家!
您可能感興趣的文章:- 詳解Golang并發(fā)操作中常見的死鎖情形
- Go 語言中的死鎖問題解決
- Go語言死鎖與goroutine泄露問題的解決
- golang coroutine 的等待與死鎖用法
- go select編譯期的優(yōu)化處理邏輯使用場景分析
- Django實(shí)現(xiàn)jquery select2帶搜索的下拉框
- Go語言使用select{}阻塞main函數(shù)介紹
- golang中的select關(guān)鍵字用法總結(jié)
- Go select 死鎖的一個(gè)細(xì)節(jié)